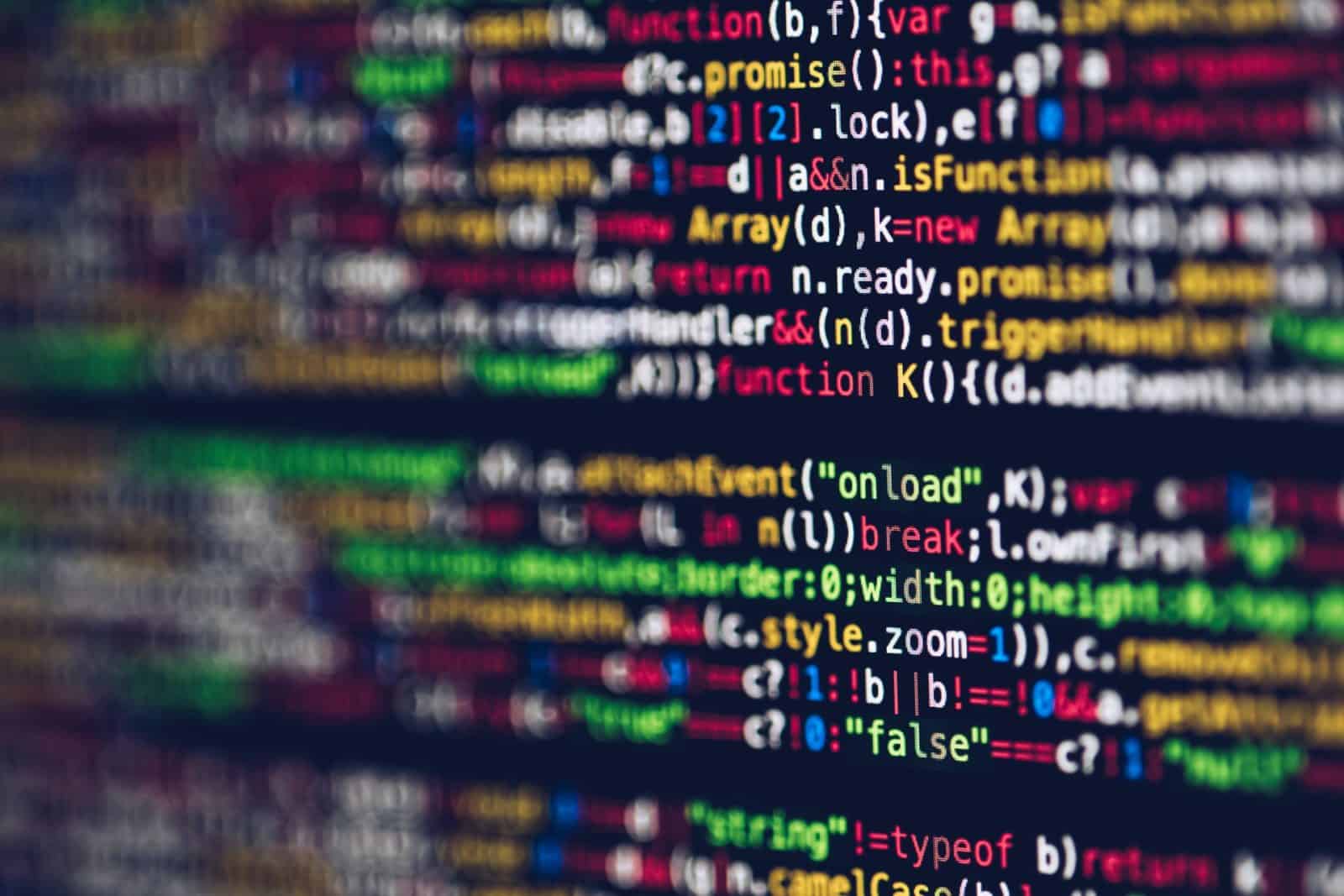
This is a really awesome script which works great for javascript applications. If you’ve saved JSON objects in the DOM, use the script below to export it into a CSV file for download. I’ve tried multiple scripts I found via Stack Overflow and such, but this one works the best:
The javascript CSV export function
function exportToCsv(filename, rows) { var processRow = function (row) { var finalVal = ''; for (var j = 0; j < row.length; j++) { var innerValue = row[j] === null ? '' : row[j].toString(); if (row[j] instanceof Date) { innerValue = row[j].toLocaleString(); }; var result = innerValue.replace(/"/g, '""'); if (result.search(/("|,|\n)/g) >= 0) result = '"' + result + '"'; if (j > 0) finalVal += ','; finalVal += result; } return finalVal + '\n'; }; var csvFile = ''; for (var i = 0; i < rows.length; i++) { csvFile += processRow(rows[i]); } var blob = new Blob([csvFile], { type: 'text/csv;charset=utf-8;' }); if (navigator.msSaveBlob) { // IE 10+ navigator.msSaveBlob(blob, filename); } else { var link = document.createElement("a"); if (link.download !== undefined) { // feature detection // Browsers that support HTML5 download attribute var url = URL.createObjectURL(blob); link.setAttribute("href", url); link.setAttribute("download", filename); link.style = "visibility:hidden"; document.body.appendChild(link); link.click(); document.body.removeChild(link); } } }
An example on how I use this function:
//example JSON object (potentially saved in the DOM) var data_in_dom = [{ 'id' : 1, 'name' : 'Anthony', 'address' : '1570 Woodward', 'city' : 'Detroit', 'region' : 'MI', 'postal_code' : '48226', 'country' : 'US', }]; //create CSV header labels var exportData = [[ 'ID', 'Name', 'Address', 'City', 'Region', 'Postal Code', 'Country']]; //prepare a JSON element for CSV export jQuery.each(data_in_dom, function(i, currentRow) { exportData.push([ currentRow.id, currentRow.name, currentRow.address, currentRow.city, currentRow.region, currentRow.postal_code, currentRow.country ]); }); //create a date/time string to be used in the file name var now = new Date(); var currentDateTimeString = now.getMonth()+1 + '-' + now.getDate() + '-' + now.getFullYear() + '-' + now.getHours() + now.getMinutes() + now.getSeconds(); //generate file exportToCsv('CustomReport-' + currentDateTimeString + '.csv', exportData);
Anthony Montalbano
If it's worth doing, it's worth doing right.
Published on: February 7, 2015
Last modified on: December 8, 2021