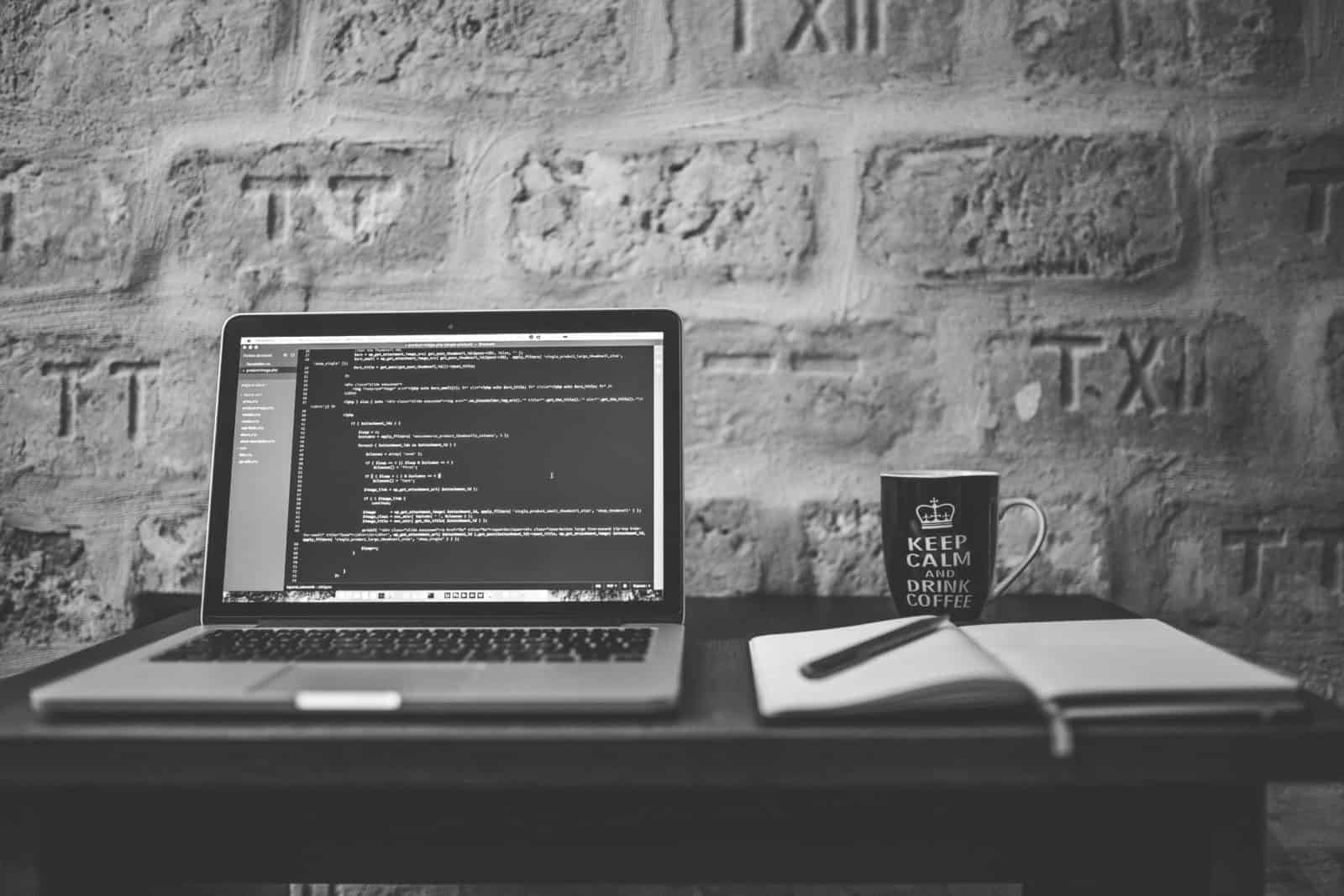
Sometimes you want to allow a css transition to finish running before executing a block of javascript. This can be easily acheived using the “transitionend” and “webkitTransitionEnd” functions. I’m going to demonstrate using jQuery, but you can use the same approach without jQuery.
CSS:
.example-17 { position: relative; display: block; width: 100%; height: 100px; background-color: grey; margin: 20px 0; } .example-17 .box { position: absolute; top: 0; left: 0; width: 25%; height: 100%; color: #fff; background-color: blue; -webkit-transition: left 2s; transition: left 2s; } .example-17 .start { left: 75%; } .example-17 .finished { background-color: red; }
Javascript:
jQuery(".example-17 .box").on("click", {}, function(evt) { jQuery(this).addClass("start"); }).on("transitionend webkitTransitionEnd", {}, function(evt) { jQuery(this).addClass("finished"); jQuery(this).text("finished"); });
Anthony Montalbano
If it's worth doing, it's worth doing right.
Published on: December 23, 2014
Last modified on: December 8, 2021